Spring Boot | Mapper에 파라미터 사용하기 | page 처리 | Annotation 매핑에서 xml을 이용한 매핑으로 변경
Mapper에 파라미터 사용하기 값을 입력 받음 page field query NoticeController.java 인터페이스가 바뀌었다. 구현체에 인자를 넣음 서비스가 가 진인자와 Dao가 가지는 인자는 1:1로 매칭 되는 경우가 많지.
kjh95.tistory.com
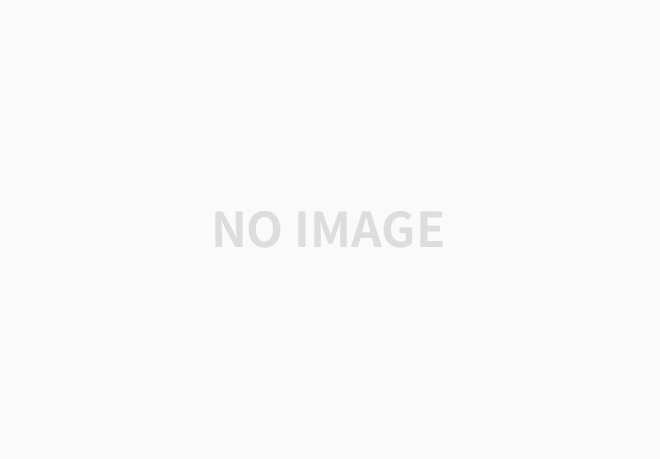
1. 서비스 구현 - 공지사항 Service 구현
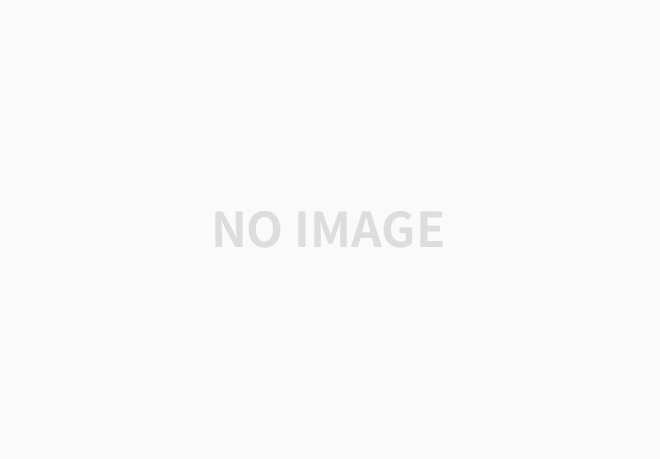
NoticeService.java
package com.newlecture.web.service;
import java.util.List;
import com.newlecture.web.entity.Notice;
import com.newlecture.web.entity.NoticeView;
public interface NoticeService {
// 페이지 요청할 때
List<NoticeView> getViewLsit();
// 검색을 요청할 때
List<NoticeView> getViewLsit(String field, String query);
// 페이지를 요청할 떄
List<NoticeView> getViewList(int page, String field, String query);
int getCount();
int getCount(String field, String query);
// 자세한 페이지를 요청할 경우
NoticeView getView(int id);
Notice getNext(int id);
Notice getPrev(int id);
// 일괄공개를 요청할 때
int updatePubAll(int[] pubIds, int[] closeIds);
// 일괄삭제를 요청할 때
int deleteAll(int[] ids);
// 수정 페이지를 요청할 경우
int update(Notice notice);
int delete(int id);
int insert(Notice notice);
}
2. Dao가 구현해야되는 목록 만들어 주기
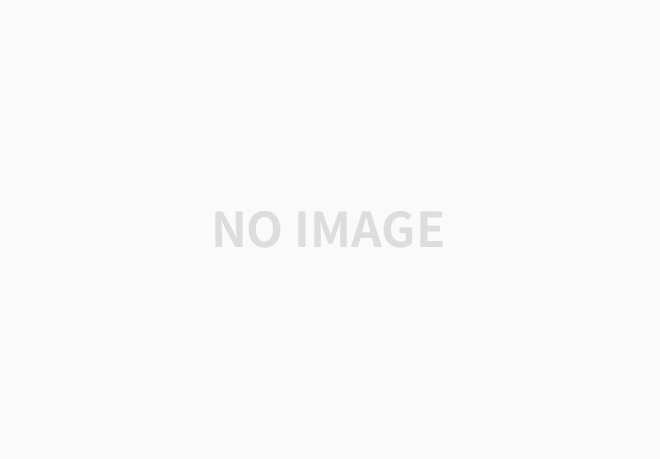
NoticeServiceImp.java
인자가 많은 메서드를 재사용하는 방식으로 집중화하기
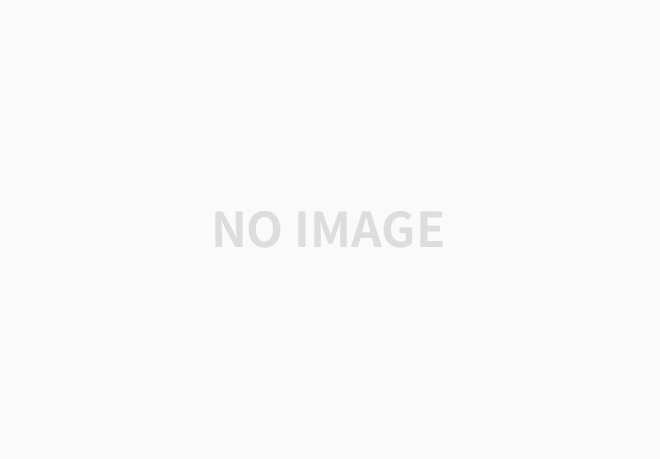
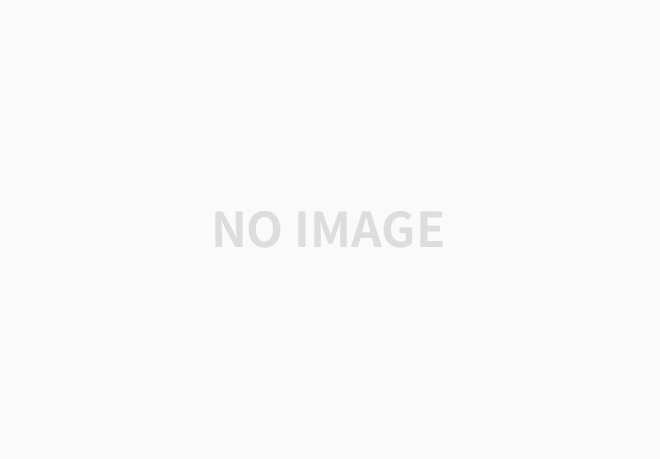
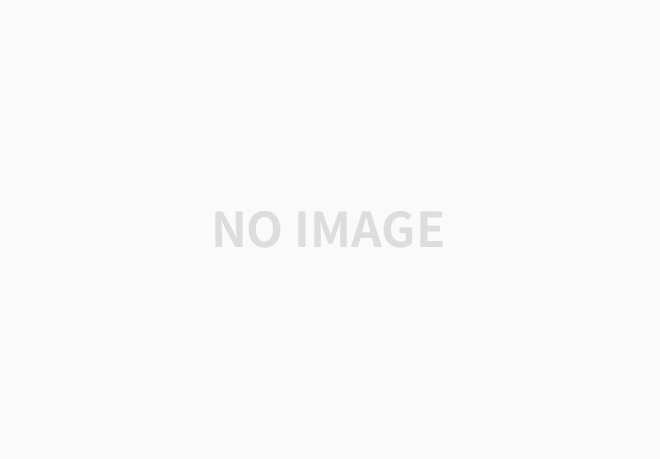
NoticeServiceImp.java
package com.newlecture.web.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.newlecture.web.dao.NoticeDao;
import com.newlecture.web.entity.Notice;
import com.newlecture.web.entity.NoticeView;
@Service
public class NoticeServiceImp implements NoticeService{
@Autowired
private NoticeDao noticeDao;
@Override
public List<NoticeView> getViewLsit() {
// TODO Auto-generated method stub
return getViewList(1, "title", "");
}
@Override
public List<NoticeView> getViewLsit(String field, String query) {
// TODO Auto-generated method stub
return getViewList(1, field, query);
}
@Override
public List<NoticeView> getViewList(int page, String field, String query) {
int size = 10;
int offset = 0+(page-1)*size; //page가 1일 경우에 offset은 0, 2->10, 3->20 패턴이 있다.(등차수열) an=a1+(n-1)d -> 0+(page-1)*10
List<NoticeView> list = noticeDao.getViewList(offset, size, field, query);
return list;
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return getCount();
}
@Override
public int getCount(String field, String query) {
// TODO Auto-generated method stub
return noticeDao.getCount(field, query);
}
@Override
public NoticeView getView(int id) {
NoticeView notice = noticeDao.getView(id);
return notice;
}
@Override
public Notice getNext(int id) {
// TODO Auto-generated method stub
return noticeDao.getNext(id);
}
@Override
public Notice getPrev(int id) {
// TODO Auto-generated method stub
return noticeDao.getPrev(id);
}
@Override
public int updatePubAll(int[] pubIds, int[] closeIds) {
// TODO Auto-generated method stub
return noticeDao.updatePubAll(pubIds, closeIds);
}
@Override
public int deleteAll(int[] ids) {
// TODO Auto-generated method stub
return noticeDao.deleteAll(ids);
}
@Override
public int update(Notice notice) {
// TODO Auto-generated method stub
return noticeDao.update(notice);
}
@Override
public int delete(int id) {
// TODO Auto-generated method stub
return noticeDao.delete(id);
}
@Override
public int insert(Notice notice) {
// TODO Auto-generated method stub
return noticeDao.insert(notice);
}
}
NoticeDao.java
package com.newlecture.web.dao;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import com.newlecture.web.entity.Notice;
import com.newlecture.web.entity.NoticeView;
@Mapper
public interface NoticeDao {
List<NoticeView> getViewList(int offset, int size, String field, String query);
int getCount(String field, String query);
NoticeView getView(int id);
Notice getNext(int id);
Notice getPrev(int id);
int updatePubAll(int[] pubIds, int[] closeIds);
int deleteAll(int[] ids);
int update(Notice notice);
int delete(int id);
int insert(Notice notice);
}
3. Dao Mapper 구현
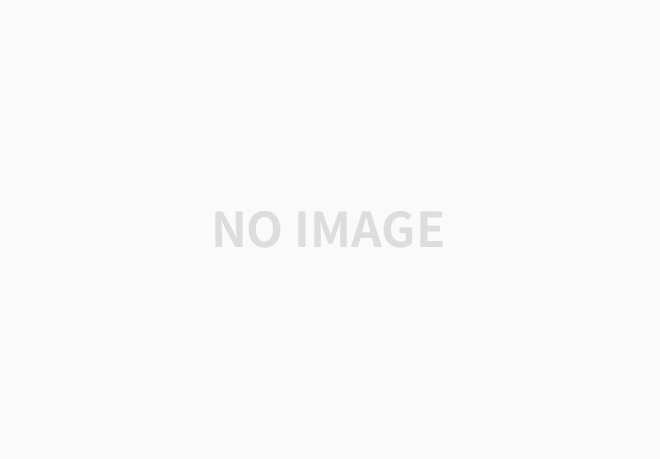
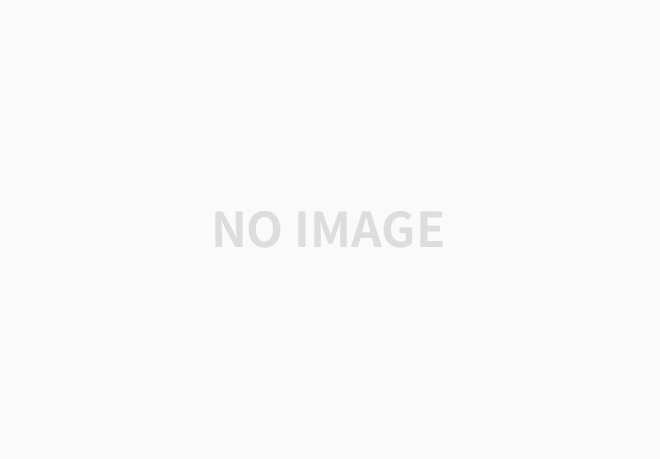
getNext는 다음번에 해당하는 id값을 반환해줘야 한다.
-> 받은 id보다 큰것에서 가장오래된 날짜의 첫번째 것을 얻으면 된다.
> -> '>' 기호
getPrev는 이전번에 해당하는 id값을 반환해줘야 한다.
< -> '<'
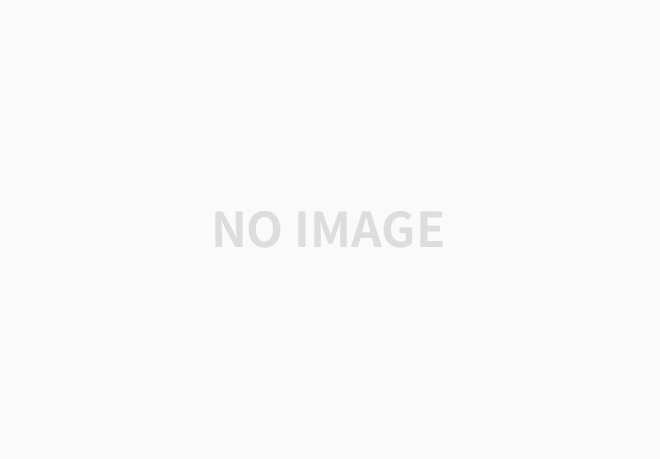
insert, update, delete 구현
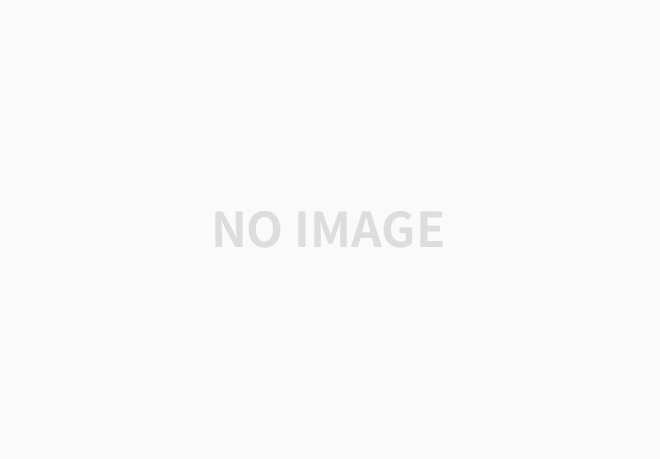
deleteAll 구현 - 정수가 여러개 있는 배열을 파라미터로 받아와야 한다.
-> 배열 사용 할시 MyBatis태그 사용 foreach
MyBatis 동적SQL
mybatis.org/mybatis-3/ko/dynamic-sql.html
MyBatis – 마이바티스 3 | 동적 SQL
동적 SQL 마이바티스의 가장 강력한 기능 중 하나는 동적 SQL을 처리하는 방법이다. JDBC나 다른 유사한 프레임워크를 사용해본 경험이 있다면 동적으로 SQL 을 구성하는 것이 얼마나 힘든 작업인지
mybatis.org
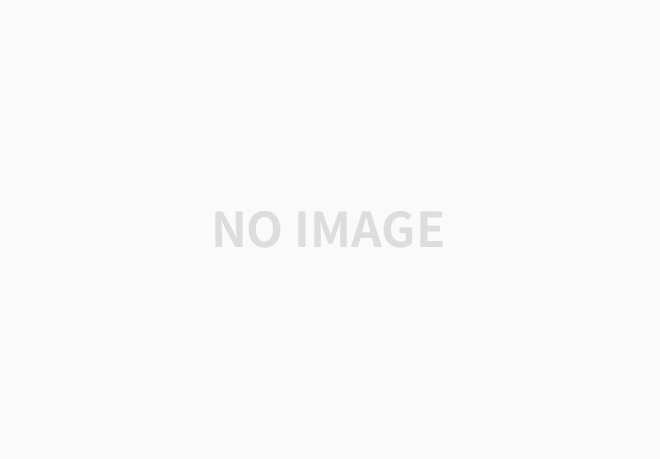
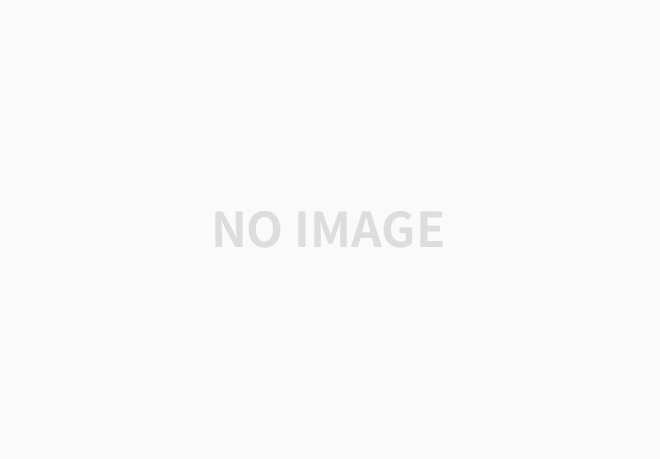
updatePubAll 구현하기 - 1방법
공개할 아이디 비공개할 아이디가 파라미터 값을 가지고 있다.
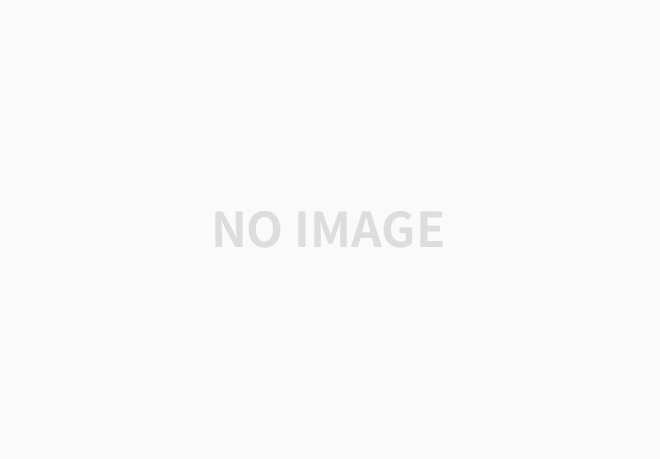
updatePubAll 하나의 배열값으로 구현 - 2방법
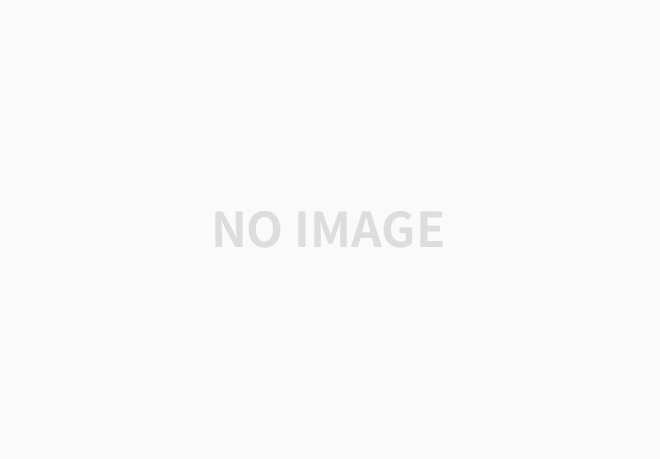
getViewList 구현
쿼리에 들어갈 내용이 대문자거나 null일 경우 조건처리
mybatis 동적 SQL문 사용
where, if
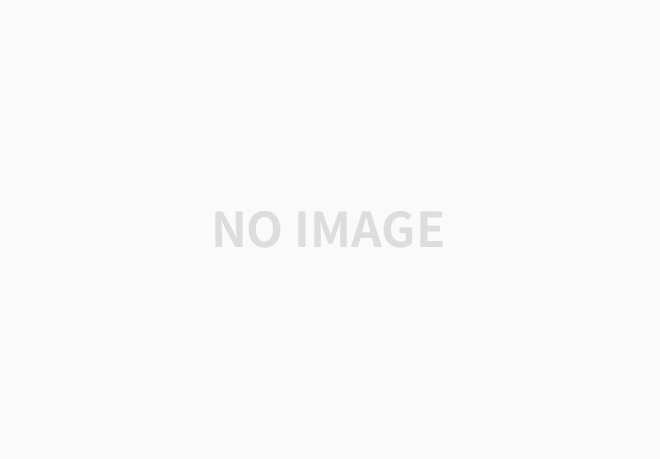
trim 사용
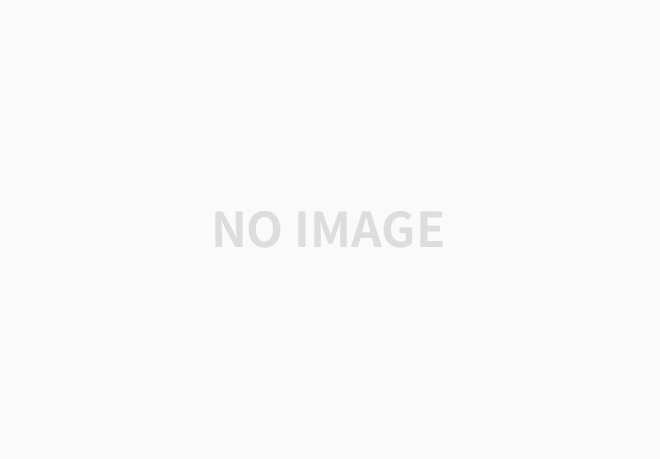
NoticeDao.java
package com.newlecture.web.dao;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import com.newlecture.web.entity.Notice;
import com.newlecture.web.entity.NoticeView;
@Mapper
public interface NoticeDao {
List<NoticeView> getViewList(int offset, int size, String field, String query, boolean pub);
int getCount(String field, String query);
NoticeView getView(int id);
Notice getNext(int id);
Notice getPrev(int id);
int update(Notice notice);
int delete(int id);
int insert(Notice notice);
int deleteAll(int[] ids);
//int updatePubAll(int[] pubIds, int[] closeIds);
int updatePubAll(int[] id, boolean pub);
}
NoticeDaoMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.newlecture.web.dao.NoticeDao">
<select id="getViewList" resultType="com.newlecture.web.entity.NoticeView">
select * from noticeview
<where>
<if test="query != null or query != ''">
${field} like '%${query}%'
</if>
and pub = #{pub}
</where>
order by regdate desc
limit #{offset}, #{size}
</select>
<select id="getCount">
select count(id) count from notice
where ${field} like '%${query}%'
</select>
<select id="getView" resultType="com.newlecture.web.entity.NoticeView">
select * from noticeview
where id=#{id]
</select>
<select id="getNext" resultType="com.newlecture.web.entity.Notice">
select * from notice
where regdate > (select regdate from notice where id = #{id})
limit 1
</select>
<select id="getPrev" resultType="com.newlecture.web.entity.Notice">
select * from notice
where regdate < (select regdate from notice where id = #{id})
order by regdate desc
limit 1
</select>
<update id="update" parameterType="com.newlecture.web.entity.Notice">
update
set
title = #{title},
content = #{content},
hit = #{hit},
pub = #{pub}
where id = #{id}
</update>
<insert id="insert" parameterType="com.newlecture.web.entity.Notice">
insert into Notice(title,content,memberId)
values(#{title},#{content},#{memberId})
</insert>
<delete id="delete">
delete from Notice
where id=#{id}
</delete>
<delete id="deleteAll">
delete from Notice
where id in
<foreach item="id" index="index" collection="ids"
open="(" separator="," close=")">
#{id}
</foreach>
</delete>
<update id="updatePubAll">
update Notice
set
pub = #{pub}
where id in
<foreach item="id" index="index" collection="ids"
open="(" separator="," close=")">
#{id}
</foreach>
<!--
<update id="updatePubAll">
update Noitce
set
pub = case id
<foreach item="id" collection="pubIds" >
when #{id} then 1
</foreach>
<foreach item="id" collection="closeIds" >
when #{id} then 0
</foreach>
end
where id in (
<foreach item="id" collection="pubIds" >
#{id}
</foreach>
,
<foreach item="id" collection="closeIds" >
#{id}
</foreach>
)
-->
<!--
update Noitce
set
pub = case id
when 14 then 0
when 15 then 0
when 21 then 1
when 22 then 1
end
where id in (14, 15, 21, 22)
-->
</update>
</mapper>