Spring Boot | Restful API 서버 만들기 | Postman 테스트
spring tool suite 4 사용 spring starter project 생성 model 만들기 UserProfile.java package com.newlecture.web.model; public class UserProfile { //서버 어플리케이션 개발에서는 멤버변수를 모두 priva..
kjh95.tistory.com
MySQL에 있는 DB정보를 연동하여 저장, 조회, 수정, 삭제
테이블 만들기
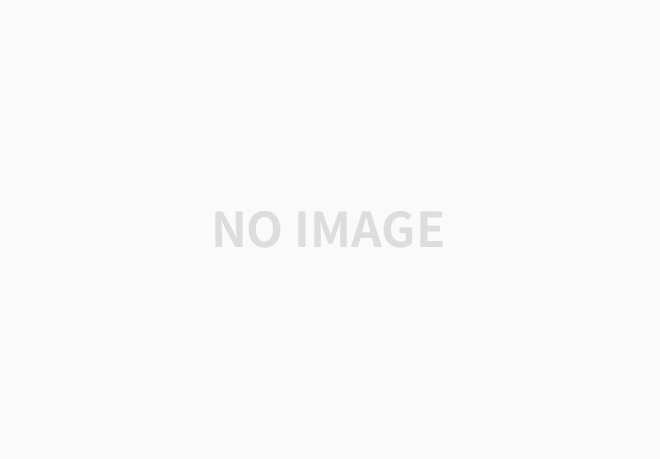
create table UserProfile (
id varchar(64) primary key,
name varchar(64),
phone varchar(64),
address varchar(256)
);
MySQL 접속 라이브러리, MyBatis 의존성 추가하기
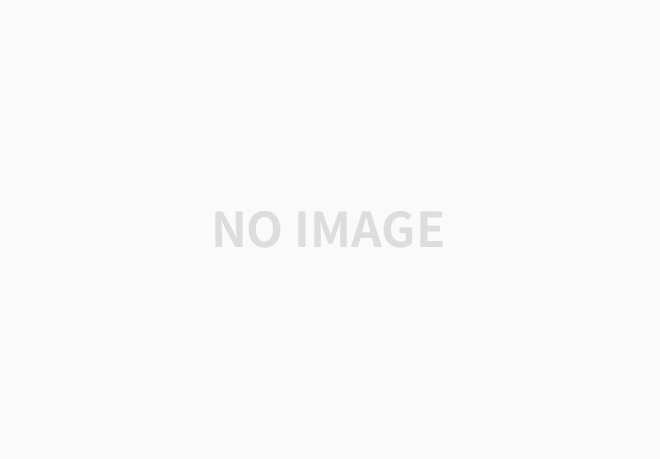
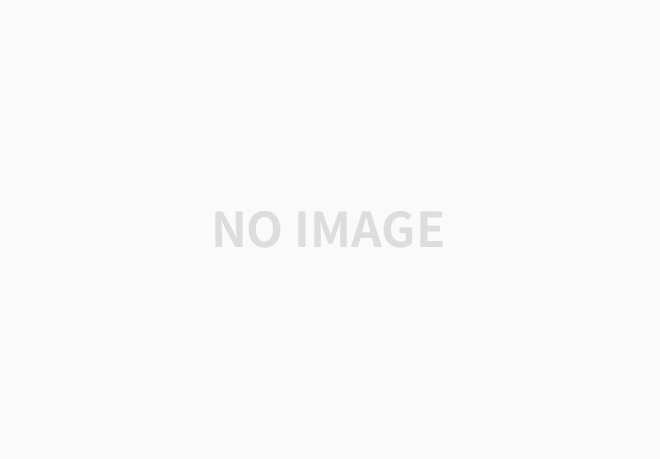
연결정보 설정하기
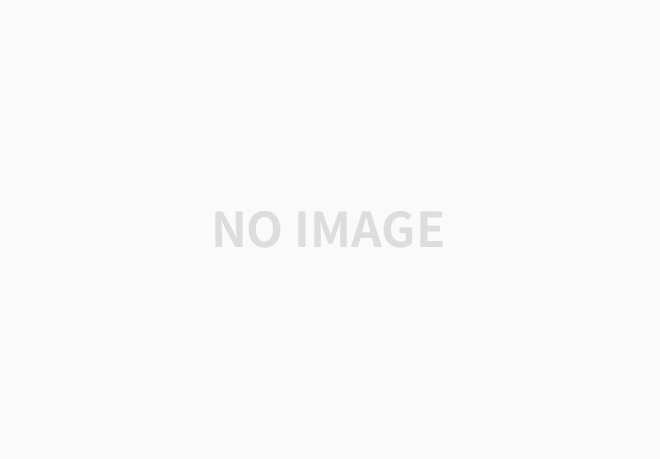
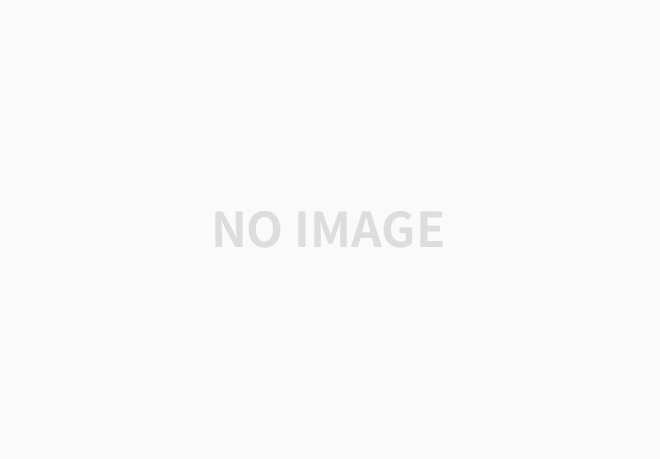
SQL Mapping
자바의 메서드와 SQL을 Mapping 해주는 기술
Mapper 만들기
패키지 생성, 인터페이스 생성
package com.newlecture.web.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import com.newlecture.web.model.UserProfile;
@Mapper
public interface UserProfileMapper {
@Select("SELECT * FROM UserProfile WHERE id=#{id}")
UserProfile getUserProfile(@Param("id") String id);
@Select("SELECT * FROM UserProfile")
List<UserProfile> getUserProfileList();
@Insert("INSERT INTO UserProfile VALUES(#{id}, #{name}, #{phone}, #{address})")
int insertUserProfile(@Param("id") String id, @Param("name") String name, @Param("phone") String phone, @Param("address") String address);
@Update("UPDATE UserProfile SET name=#{name}, phone=#{phone}, address=#{address} WHERE id=#{id}")
int updateUserProfile(@Param("id") String id, @Param("name") String name, @Param("phone") String phone, @Param("address") String address);
@Delete("DELETE FROM UserProfile WHERE id=#{id}")
int deleteUserProfile(@Param("id") String id);
}
컨트롤러에서 Mapper 사용하기
생성자 만들기
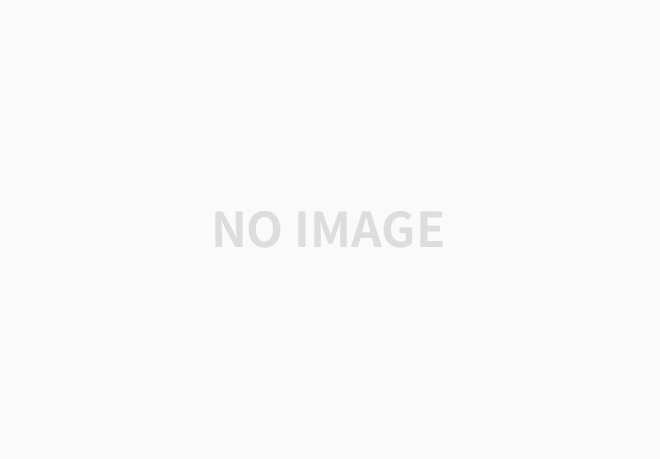
리턴값 바꿔주기
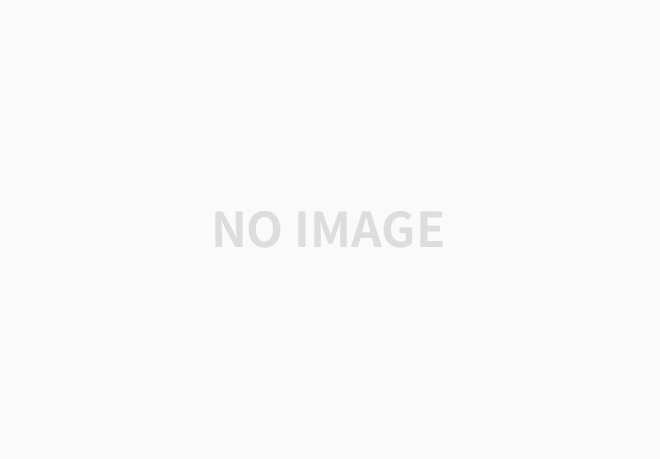
UserProfileController.java
package com.newlecture.web.controller;
import java.util.List;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.newlecture.web.mapper.UserProfileMapper;
import com.newlecture.web.model.UserProfile;
//스프링이 알아서 컨트롤러로 인식하는 어노테이션
@RestController
public class UserProfileController {
private UserProfileMapper mapper;
public UserProfileController(UserProfileMapper mapper) {
this.mapper = mapper;
}
//UerProfile 이라는 객체를 리턴하면 이 객체를 json형태로 자동으로 리턴해서 클라이언트에 전달.
@GetMapping("/user/{id}")
public UserProfile getUserProfile(@PathVariable("id") String id) {
return mapper.getUserProfile(id);
}
@GetMapping("/user/all")
public List<UserProfile> getUserProfileList() {
return mapper.getUserProfileList();
}
//추가할 아이디 이름 주소를 파라미터로 전달받는다.
@PutMapping("/user/{id}")
public void putUserProfile(@PathVariable("id") String id, @RequestParam("name") String name, @RequestParam("phone") String phone, @RequestParam("address") String address) {
mapper.insertUserProfile(id, name, phone, address);
}
//수정
@PostMapping("/user/{id}")
public void postUserProfile(@PathVariable("id") String id, @RequestParam("name") String name, @RequestParam("phone") String phone, @RequestParam("address") String address) {
mapper.updateUserProfile(id, name, phone, address);
}
//삭제
@DeleteMapping("/user/{id}")
public void deleteUserProfile(@PathVariable("id") String id) {
mapper.deleteUserProfile(id);
}
}
Postman 사용해서 테스트하기
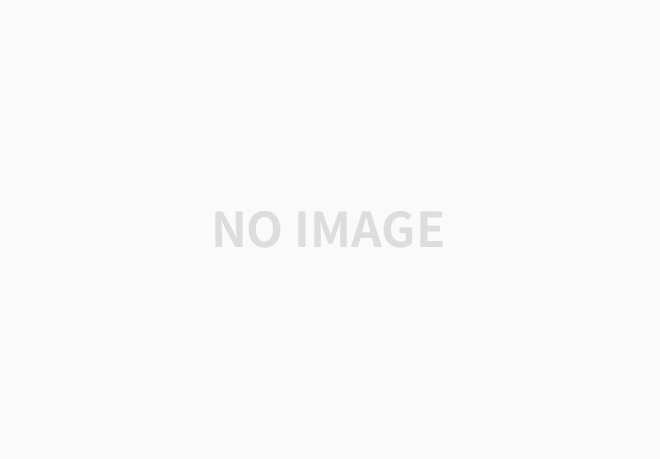
● 아이디 2번으로 PUT 테스트
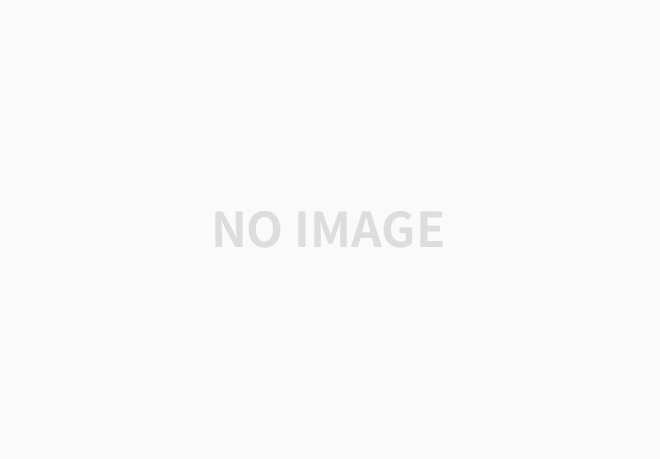
● 전체 리스트 출력 테스트
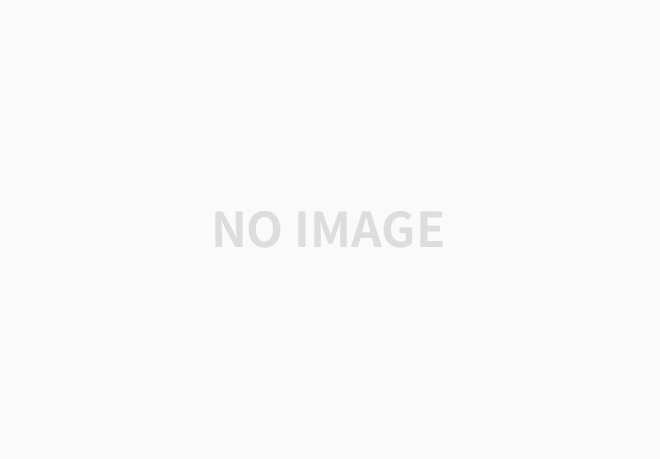
● 삭제 테스트
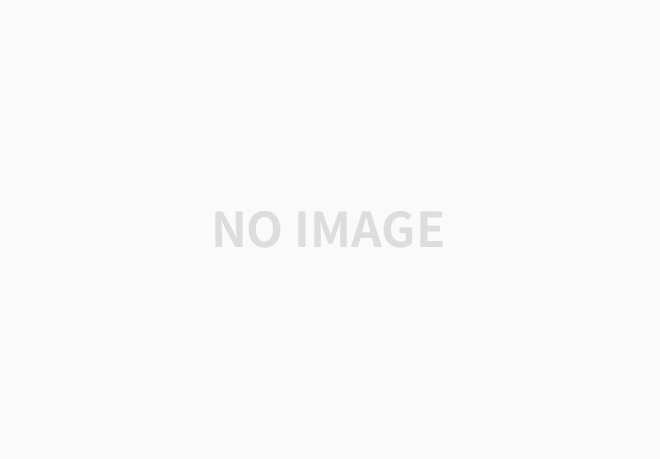
● 수정 테스트
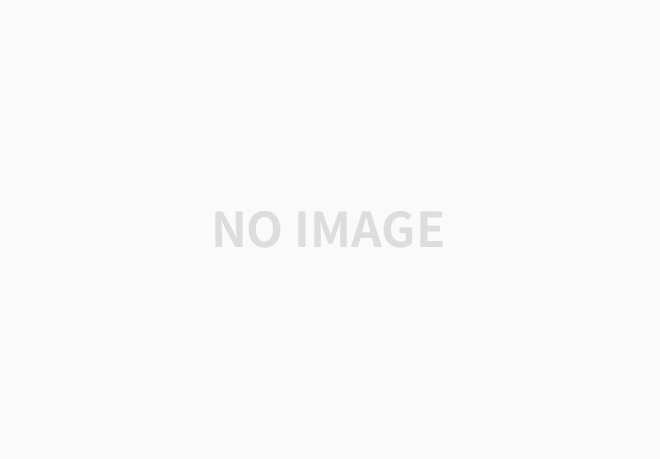
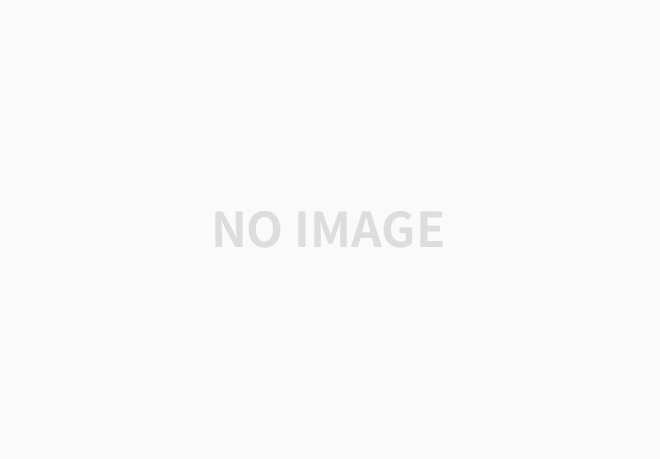
MySQL Workbench 확인
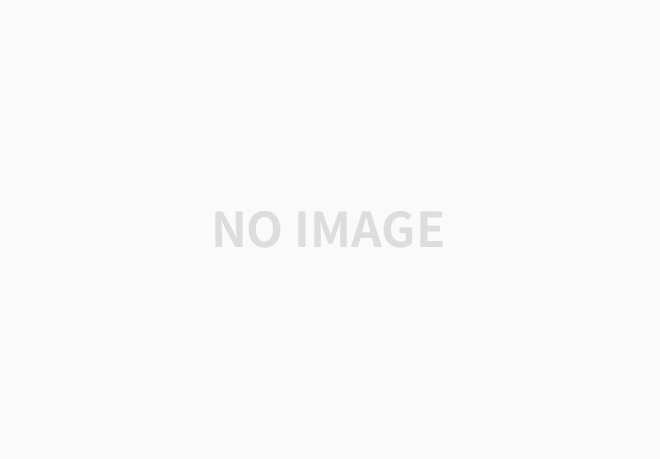